Code Snippets
Move elements at different breakpoints
Move elements at different breakpoints
A useful javascript snippet that lets you move elements to a new location at different breakpoints. Perfect if you can only have 1 instance of an element on the page but it needs to change position.
<script>
/*
This code is used to append items to different divs at different breakpoints.
It allows us to be more consistent with the development and align it to the design
without back and forth from designers.
*/
function swap(breakpoint, element, location) {
if (window.innerWidth < breakpoint) {
// Append 'location' to 'element' if the current window width is less than 'breakpoint'
element.appendChild(location);
} else {
// Log an error if 'element' or 'location' are not valid HTMLElements
console.error('Invalid element or location');
}
}
</script>
Similar Code Snippets

Custom Text Selection Colour
When users highlight text on your webpage, you have a unique opportunity to enhance the visual feedback with custom colors that match your brand. The ::selection pseudo-element allows you to style the background and text color of the selected text:
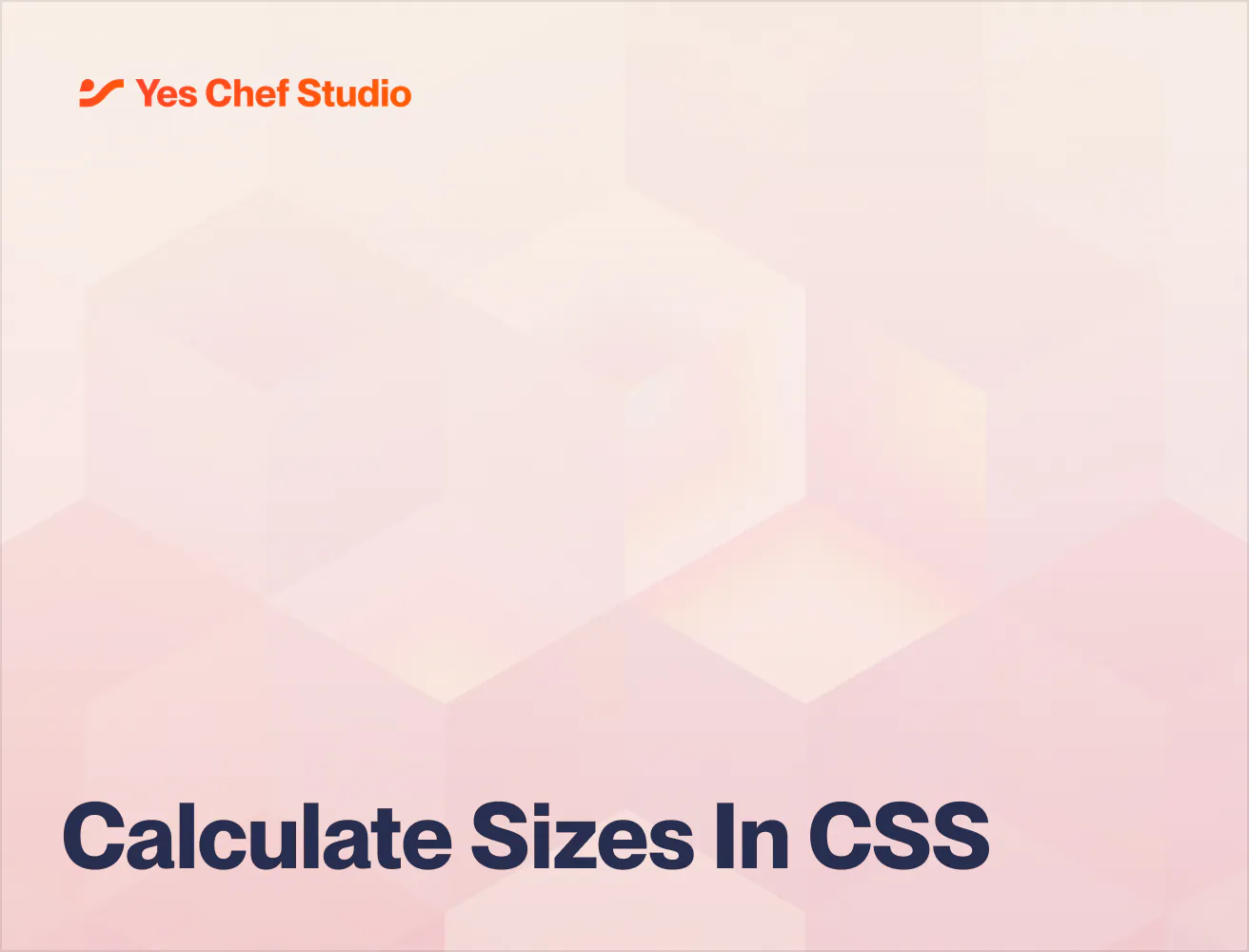
Calculate sizes in CSS
CSS's calc() function allows you to perform calculations to determine CSS property values. It's incredibly useful for responsive design, such as when you need to adjust sizes dynamically. For example, if you want a div to be 100% of the viewport width minus a certain margin, you can use calc():

Fix side scrolling on all devices
The .page-wrapper is a common class used to wrap the entire content of a page. Sometimes, you might encounter elements that overflow the boundaries of your page, causing horizontal scrollbars or layout shifts. To address this, you can use the overflow: clip; property to prevent any content from overflowing the boundaries of the .page-wrapper: