Code Snippets
Pull content from another page
Pull content from another page
404 page need a collection list? Webflow told you it can't happen? We've got you covered. Use this code to pull in the nav or entire page design from another page into your 404.
<script>
/**
* Fetches HTML content from a specified URL and appends a selected element from it to the current document.
* Useful for dynamically loading and displaying content from another part of your website.
*/
async function fetchAndAppendElement() {
try {
// Specify the URL from which to fetch HTML content. Adjust '/' to your specific needs.
const response = await fetch('/');
// Check if the fetch request was successful
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
// Extract the HTML text from the response
const html = await response.text();
// Create a temporary container to parse the fetched HTML text
const container = document.createElement('div');
container.innerHTML = html;
// Adjust the selector below to target the specific element you want to fetch from the fetched content
const fetchedElement = container.querySelector("[element-ajax='content']");
if (fetchedElement) {
// Adjust the selector below to target where in the current document you want to append the fetched element
const appendItem = document.querySelector("[element-ajax='wrapper']");
// Clone the fetched element to prevent modifying the original element in the temporary container
const clonedElement = fetchedElement.cloneNode(true);
// Append the cloned element. Ensure appendItem is not null before appending.
if (appendItem) {
appendItem.appendChild(clonedElement);
}
} else {
console.error('Failed to fetch the element. Check the selector for the element you are trying to fetch.');
}
} catch (error) {
console.error('An error occurred during the fetch and append operation:', error);
}
}
/**
* Calls fetchAndAppendElement function when the page has finished loading.
* This ensures the DOM is ready for manipulation.
*/
window.addEventListener('DOMContentLoaded', fetchAndAppendElement);
</script>
Similar Code Snippets

Fix side scrolling on all devices
The .page-wrapper is a common class used to wrap the entire content of a page. Sometimes, you might encounter elements that overflow the boundaries of your page, causing horizontal scrollbars or layout shifts. To address this, you can use the overflow: clip; property to prevent any content from overflowing the boundaries of the .page-wrapper:
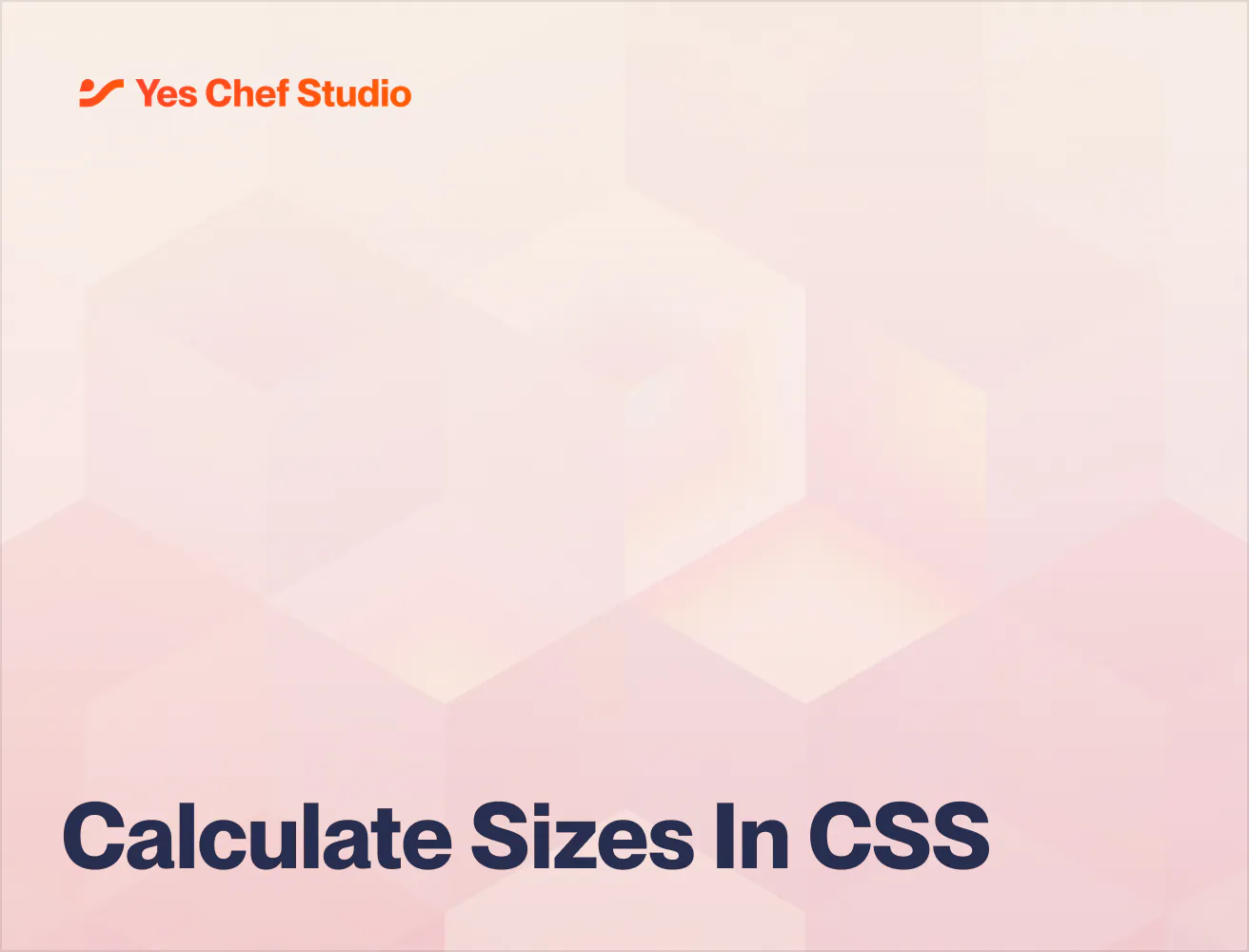
Calculate sizes in CSS
CSS's calc() function allows you to perform calculations to determine CSS property values. It's incredibly useful for responsive design, such as when you need to adjust sizes dynamically. For example, if you want a div to be 100% of the viewport width minus a certain margin, you can use calc():

Balance text wrapping
Headings are crucial for grabbing attention and conveying key messages. However, unbalanced text lines can make headings less impactful. The text-wrap: balance; property helps distribute text more evenly across lines, reducing the occurrence of "widows" - a single word or short line at the end of a paragraph: