Code Snippets
Add query parameter value to hidden field
Add query parameter value to hidden field
Pass through dynamic url values to your forms so you can track things like sales reps, traffic source, etc....
<script>
// Listen for when the HTML document has been completely loaded
document.addEventListener('DOMContentLoaded', function() {
// Function to get a specific query parameter value from the URL
function getQueryParamValue(paramName) {
// Create an object to parse the URL query parameters
const params = new URLSearchParams(window.location.search);
// Return the value of the query parameter (e.g., 'salesRep')
return params.get(paramName);
}
// Call the function to get the 'salesRep' query parameter value
var queryValue = getQueryParamValue('your_query_parameter'); // change to your query parameter name
// Check if the query parameter exists
if (!queryValue) {
// Exit if the parameter is not present in the URL
return;
}
// Get all forms on the current web page
var forms = document.querySelectorAll('form');
// Loop through each form found
forms.forEach(function(form) {
// Find a specific hidden field within the form
var hiddenField = form.querySelector('#your_field_id[type="hidden"]'); // Update selector as needed
// If the hidden field is found, set its value
if (hiddenField) {
hiddenField.value = queryValue;
}
});
});
</script>
Similar Code Snippets

Fix side scrolling on all devices
The .page-wrapper is a common class used to wrap the entire content of a page. Sometimes, you might encounter elements that overflow the boundaries of your page, causing horizontal scrollbars or layout shifts. To address this, you can use the overflow: clip; property to prevent any content from overflowing the boundaries of the .page-wrapper:
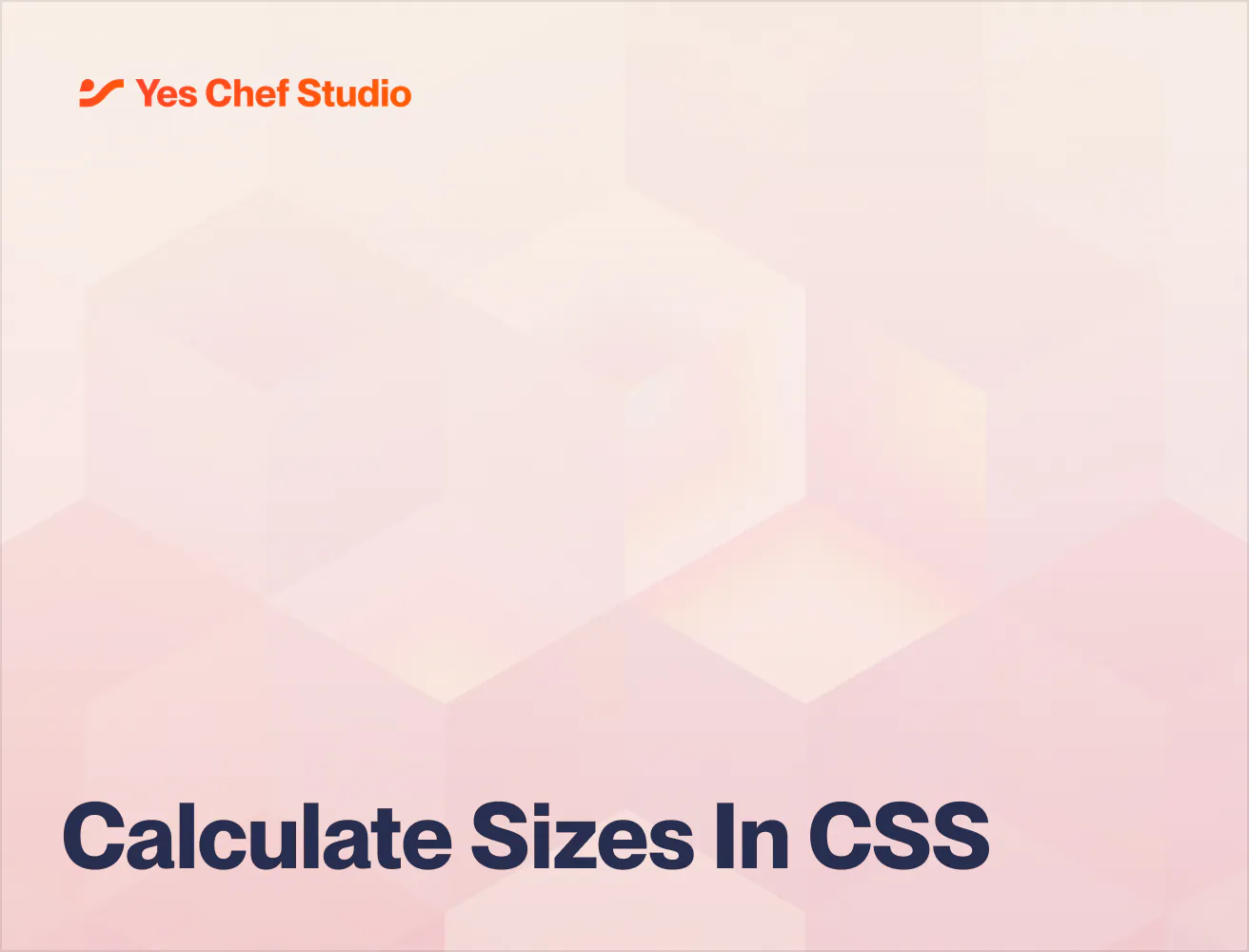
Calculate sizes in CSS
CSS's calc() function allows you to perform calculations to determine CSS property values. It's incredibly useful for responsive design, such as when you need to adjust sizes dynamically. For example, if you want a div to be 100% of the viewport width minus a certain margin, you can use calc():

Balance text wrapping
Headings are crucial for grabbing attention and conveying key messages. However, unbalanced text lines can make headings less impactful. The text-wrap: balance; property helps distribute text more evenly across lines, reducing the occurrence of "widows" - a single word or short line at the end of a paragraph: